Manage users
You can use the APIv2 to integrate an IXON Cloud user invite in your own online environment. you can also add a list of users, or remove a user using the APIv2. After you have requested the basic information headers, you only need three API requests to complete this action.
User management
To comply with IXONs new user management system, you first need to get the ID's for the role and group you'd like to add a new user to. You can do that using the following request for the role ID. The endpoint is RoleList.
curl --request GET \
--url '<<url>>:443/api/roles?fields=publicId,name' \
--header 'Api-Version: 2' \
--header "Api-Application: $application_id" \
--header "Api-Company: $company_id" \
--header 'Content-Type: application/json' \
--header "Authorization: Bearer $bearer_token"
To obtain a group ID, you have to use the following request. When you want to assign a user to a company wide role, which has access to all devices you have to use the company group, of which te group ID will be included in the response. The endpoint is GroupList.
curl --request GET \
--url '<<url>>:443/api/groups?fields=publicId,name' \
--header 'Api-Version: 2' \
--header "Api-Application: $application_id" \
--header "Api-Company: $company_id" \
--header 'Content-Type: application/json' \
--header "Authorization: Bearer $bearer_token"
User access overview
This UML diagram below shows the relationships between Role, Membership, Audience, Group, etc.
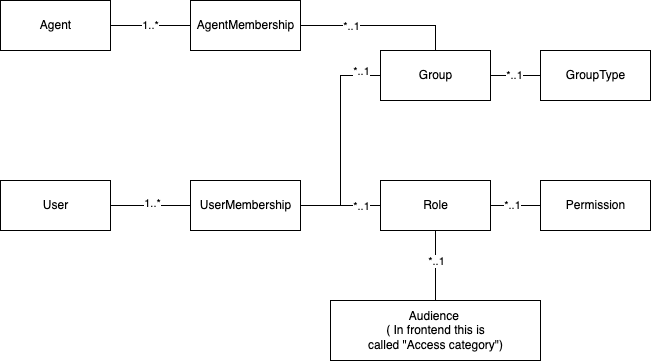
Memberships are used to determine which machines a user has access to.
Audiences are used to determine which services a user has access to on that machine.
You can use the following endpoints:
- AgentList
- AgentMembershipList
- GroupList
- GroupTypeList
- UserList
- UserMembershipList
- RoleList
- PermissionList
- AudienceList
Some remarks:
- For company-wide memberships, the group has a boolean: isCompanyGroup which is set to true;
- For device-specific access, the group has a value for the agent property that links it directly to the agent without using agentMembership. This means one group per user and device they have device-specific access to;
- AgentMembership is only used for company-wide and group-specific access.
Invite a user
Now that you have the role ID and group ID, you can send the POST
request to the InviteList endpoint to invite a new user. Below you can find an example of how that request should look. The Api-Branding header should contain the URL of your company. The default is portal.ixon.cloud, but the entered URL should be different if you use White Label Premium.
You also have to include a data field with the role ID, group ID, the email address of the person you'd like to invite. You can optionally add a message that will be included in the invite email as well.
curl --request POST \
--url '<<url>>:443/api/invites' \
--header 'Api-Version: 2' \
--header "Api-Application: $application_id" \
--header "Api-Company: $company_id" \
--header 'Content-Type: application/json' \
--header 'Api-Branding: <<apiBranding>>' \
--header "Authorization: Bearer $bearer_token" \
--data '[
{
"groupRoles": [
{
"group": {
"publicId": "$group_id"
},
"role": {
"publicId": "$role_id"
}
}
],
"emailAddress": "$email_address",
"message": "$message"
}
]'
A successful response to the request will look like the example below. An email with the invite link will be sent to the entered email address. That person can create an account to the IXON Cloud by clicking on the link if he or she currently doesn't have an IXON Cloud account.
{
"status": "success",
"type": "InviteResponse",
"data": {
"invites": [
{
"publicId": "$invite_id"
}
],
"userMemberships": [
{
"publicId": "$member_id"
}
]
}
}
Get a list of users
You can also keep a list of the users in your company in your own administration. To obtain that list you have to send a GET
request to the UserList endpoint. You can find an example of this request below.
curl --request GET \
--url '<<url>>:443/api/users' \
--header 'Api-Version: 2' \
--header "Api-Application: $application_id" \
--header "Api-Company: $company_id" \
--header 'Content-Type: application/json' \
--header "Authorization: Bearer $bearer_token"
Remove a user
The UserList endpoint can be used to remove a user as well. You first have to obtain the User ID with the GET request in the example above. You then have to add that User ID to the DELETE
request. An example of that request is given below.
curl --request DELETE \
--url '<<url>>:443/api/users' \
--header 'Api-Version: 2' \
--header "Api-Application: $application_id" \
--header "Api-Company: $company_id" \
--header 'Content-Type: application/json' \
--header "Authorization: Bearer $bearer_token" \
--data '[{"$public_id": "$user_id"}]'
Updated 3 months ago